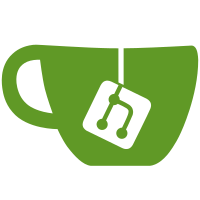
The current "allow_markup" setting will simply strip any markup from the final notification, which includes formatting elements. On top of that, literal [<>&..] symbols are not quoted before are being passed onto pango in several places, resulting in stray error messages. This patch fixes allow_markup to correctly strip markup only from the incoming notification, not from the format. You might also want to treat incoming messages as literal text (supplied by un-aware programs), in which case you need to properly quote the text before it's processed by pango. A new setting is introduced, called "plain_text", which forces incoming messages to be treated literally. allow_markup/plain_text are complimentary to each other. The new rule actions allow to narrow down the handling to a specific block, achieving notification Zen. The following is done in this patch: - Fix ruleset initialization in config.def.h. - Introduce new allow_markup/plain_text actions in the rules. - Fix handling of allow_markup to strip markup from summary/body only, preserving format's markup. - Fix broken string functions (string_replace_all didn't handle recursive replacements correctly). - Fix quoting of other literal fields (icon name/appname). - Fix handling of ignore_newline as well (applied only on summary/body). - Dunstrc update with the same previous defaults.
59 lines
1.7 KiB
C
59 lines
1.7 KiB
C
/* copyright 2013 Sascha Kruse and contributors (see LICENSE for licensing information) */
|
|
#pragma once
|
|
|
|
#include "x.h"
|
|
|
|
#define LOW 0
|
|
#define NORM 1
|
|
#define CRIT 2
|
|
|
|
typedef struct _actions {
|
|
char **actions;
|
|
char *dmenu_str;
|
|
gsize count;
|
|
} Actions;
|
|
|
|
typedef struct _notification {
|
|
char *appname;
|
|
char *summary;
|
|
char *body;
|
|
char *icon;
|
|
char *msg; /* formatted message */
|
|
char *category;
|
|
char *text_to_render;
|
|
const char *format;
|
|
char *dbus_client;
|
|
time_t start;
|
|
time_t timestamp;
|
|
int timeout;
|
|
int urgency;
|
|
bool allow_markup;
|
|
bool plain_text;
|
|
bool redisplayed; /* has been displayed before? */
|
|
int id;
|
|
int dup_count;
|
|
int displayed_height;
|
|
const char *color_strings[2];
|
|
bool first_render;
|
|
|
|
int progress; /* percentage + 1, 0 to hide */
|
|
int line_count;
|
|
const char *script;
|
|
char *urls;
|
|
Actions *actions;
|
|
} notification;
|
|
|
|
int notification_init(notification * n, int id);
|
|
void notification_free(notification * n);
|
|
int notification_close_by_id(int id, int reason);
|
|
int notification_cmp(const void *a, const void *b);
|
|
int notification_cmp_data(const void *a, const void *b, void *data);
|
|
void notification_run_script(notification * n);
|
|
int notification_close(notification * n, int reason);
|
|
void notification_print(notification * n);
|
|
char *notification_strip_markup(char *str);
|
|
char *notification_quote_markup(char *str);
|
|
void notification_update_text_to_render(notification *n);
|
|
int notification_get_ttl(notification *n);
|
|
int notification_get_age(notification *n);
|