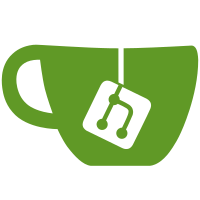
Currently triggers only the latest notification. TODO: implement it either with ActionsAll or more favorable: with an optional paramter, which should trigger only the action of a single notification. The problem currently: I have got no ability to check the DBus docs, as I'm on a bad internet connection.
95 lines
3.0 KiB
Bash
Executable File
95 lines
3.0 KiB
Bash
Executable File
#!/bin/sh
|
|
|
|
set -eu
|
|
|
|
DBUS_NAME="org.freedesktop.Notifications"
|
|
DBUS_PATH="/org/freedesktop/Notifications"
|
|
DBUS_IFAC_DUNST="org.dunstproject.cmd0"
|
|
DBUS_IFAC_PROP="org.freedesktop.DBus.Properties"
|
|
DBUS_IFAC_FDN="org.freedesktop.Notifications"
|
|
|
|
function die(){ printf "%s\n" "${1}" >&2; exit 1; }
|
|
|
|
function show_help() {
|
|
cat <<-EOH
|
|
Usage: dunstctl <command> [parameters]"
|
|
Commands:
|
|
close Close the last notification
|
|
close-all Close the all notifications
|
|
context Open context menu of latest notification
|
|
history-pop Pop one notification from history
|
|
status Check if
|
|
status-set [true|false] Set the status
|
|
debug Print debugging information
|
|
help Show this help
|
|
EOH
|
|
}
|
|
|
|
function method_call() {
|
|
dbus-send --print-reply=literal --dest="${DBUS_NAME}" "${DBUS_PATH}" $*
|
|
}
|
|
|
|
function property_get() {
|
|
dbus-send --print-reply=literal --dest="${DBUS_NAME}" "${DBUS_PATH}" "${DBUS_IFAC_PROP}.Get" "string:${DBUS_IFAC_DUNST}" "string:${1}"
|
|
}
|
|
|
|
function property_set() {
|
|
dbus-send --print-reply=literal --dest="${DBUS_NAME}" "${DBUS_PATH}" "${DBUS_IFAC_PROP}.Set" "string:${DBUS_IFAC_DUNST}" "string:${1}" "${2}"
|
|
}
|
|
|
|
command -v dbus-send >/dev/null 2>/dev/null || \
|
|
die "Command dbus-send not found"
|
|
|
|
|
|
case "${1:-}" in
|
|
"action")
|
|
method_call "${DBUS_IFAC_DUNST}.NotificationAction" "int32:${2:-0}" >/dev/null
|
|
;;
|
|
"close")
|
|
method_call "${DBUS_IFAC_DUNST}.NotificationCloseLast" >/dev/null
|
|
;;
|
|
"close-all")
|
|
method_call "${DBUS_IFAC_DUNST}.NotificationCloseAll" >/dev/null
|
|
;;
|
|
"context")
|
|
method_call "${DBUS_IFAC_DUNST}.ContextMenuCall" >/dev/null
|
|
;;
|
|
"history-pop")
|
|
method_call "${DBUS_IFAC_DUNST}.NotificationShow" >/dev/null
|
|
;;
|
|
"status")
|
|
property_get running | ( read _ _ status; printf "%s\n" "${status}"; )
|
|
;;
|
|
"status-set")
|
|
[ "${2:-}" ] \
|
|
|| die "No status parameter specified. Please give either 'true' or 'false' as running parameter."
|
|
[ "${2}" == "true" ] || [ "${2}" == "false" ] \
|
|
|| die "Please give either 'true' or 'false' as running parameter."
|
|
property_set running variant:boolean:"${2}"
|
|
;;
|
|
"help"|"--help"|"-h")
|
|
show_help
|
|
;;
|
|
"debug")
|
|
dbus-send --print-reply=literal --dest="${DBUS_NAME}" "${DBUS_PATH}" "${DBUS_IFAC_FDN}.GetServerInformation" >/dev/null 2>/dev/null \
|
|
|| die "Dunst is not running."
|
|
|
|
dbus-send --print-reply=literal --dest="${DBUS_NAME}" "${DBUS_PATH}" "${DBUS_IFAC_FDN}.GetServerInformation" \
|
|
| (
|
|
read name vendor version protocol_version
|
|
[ "${name}" == dunst ]
|
|
printf "dunst version: %s\n" "${version}"
|
|
) \
|
|
|| die "Another notification manager is running. It's not dunst"
|
|
|
|
dbus-send --print-reply=literal --dest="${DBUS_NAME}" "${DBUS_PATH}" "${DBUS_IFAC_DUNST}.Ping" >/dev/null 2>/dev/null \
|
|
|| die "Dunst controlling interface not available. Is the version too old?"
|
|
;;
|
|
"")
|
|
die "dunstctl: No command specified. Please consult the usage."
|
|
;;
|
|
*)
|
|
die "dunstctl: unrecognized command '${1:-}'. Please consult the usage."
|
|
;;
|
|
esac
|