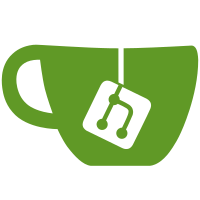
This commit adds an output struct which abstracts the X11 specific functions and makes it possible to easily create a drop-in wayland output. It also fixes a bug in wayland where notifications won't disappear. This is because wayland doesn't give access to user input when a client is not in focus. This way it seems like the user is always idle. The idle functionality is now disabled in Wayland until proper support is added.
40 lines
1.0 KiB
C
40 lines
1.0 KiB
C
/* copyright 2013 Sascha Kruse and contributors (see LICENSE for licensing information) */
|
|
#ifndef DUNST_SCREEN_H
|
|
#define DUNST_SCREEN_H
|
|
|
|
#include <stdbool.h>
|
|
#include <X11/Xlib.h>
|
|
|
|
#define INRECT(x,y,rx,ry,rw,rh) ((x) >= (rx) && (x) < (rx)+(rw) && (y) >= (ry) && (y) < (ry)+(rh))
|
|
|
|
void init_screens(void);
|
|
void screen_dpi_xft_cache_purge(void);
|
|
bool screen_check_event(XEvent *ev);
|
|
|
|
const struct screen_info *get_active_screen(void);
|
|
double screen_dpi_get(const struct screen_info *scr);
|
|
|
|
/**
|
|
* Find the currently focused window and check if it's in
|
|
* fullscreen mode
|
|
*
|
|
* @see window_is_fullscreen()
|
|
* @see get_focused_window()
|
|
*
|
|
* @retval true: the focused window is in fullscreen mode
|
|
* @retval false: otherwise
|
|
*/
|
|
bool have_fullscreen_window(void);
|
|
|
|
/**
|
|
* Check if window is in fullscreen mode
|
|
*
|
|
* @param window the x11 window object
|
|
* @retval true: \p window is in fullscreen mode
|
|
* @retval false: otherwise
|
|
*/
|
|
bool window_is_fullscreen(Window window);
|
|
|
|
#endif
|
|
/* vim: set ft=c tabstop=8 shiftwidth=8 expandtab textwidth=0: */
|